Prometheus
Oodle is a drop-in replacement for Prometheus when it comes to storing and querying metrics. It natively supports Prometheus remote write protocol and can be integrated easily with existing Prometheus-based infrastructure.
Remote Write Configuration
In the Oodle UI, navigate to Settings
page and click on Connect
in the Prometheus
tile.
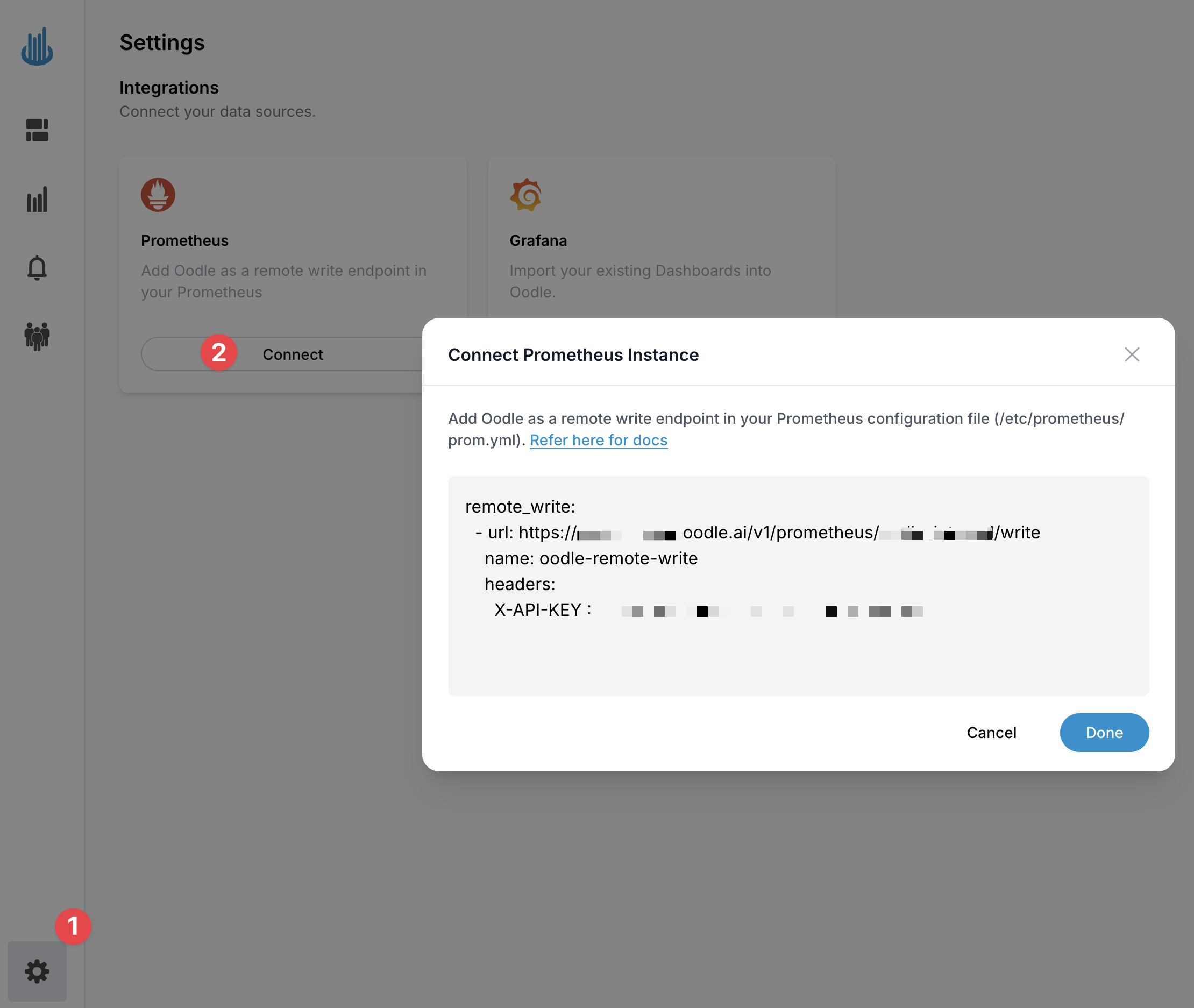
It provides you with the remote_write
configuration that you can use in your Prometheus configuration
which will be pre-populated with the values specific to your Oodle instance.
remote_write:
- url: https://<OODLE_ENDPOINT>/v1/prometheus/<INSTANCE_ID>/write
name: oodle-remote-write
headers:
X-API-KEY: <API_KEY>
After adding the remote write configuration, you need to restart your Prometheus service
to apply the changes. Once Oodle starts receiving the metrics, the status of the Prometheus
tile will change to Receiving
state with a green checkmark.
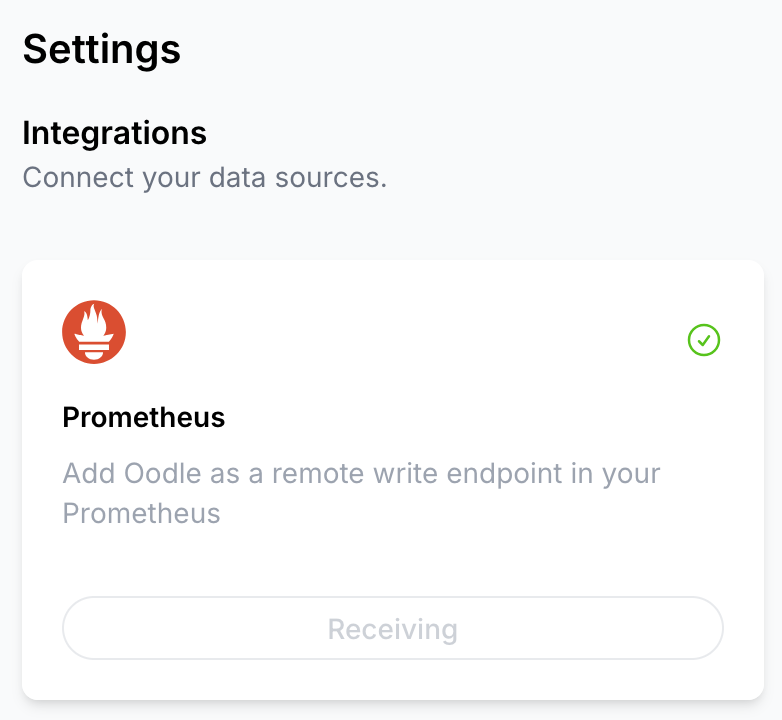
Victoria Metrics Agent (vmagent
)
If you are using Victoria Metrics Agent (vmagent
) to scrape metrics from your targets, you can
configure it to send metrics to Oodle by adding the -remoteWrite.url
and -remoteWrite.headers
flags to the vmagent
command line.
-remoteWrite.url=https://<OODLE_ENDPOINT>/v1/prometheus/<INSTANCE_ID>/write
-remoteWrite.headers='X-API-KEY: <API_KEY>'
Visualize Metrics
You can visualize metrics in Oodle using the Explore Metrics
tab. It supports Grafana
Builder and Code Editor to build PromQL queries. Please refer to
PromQL Query docs
to learn more about PromQL.
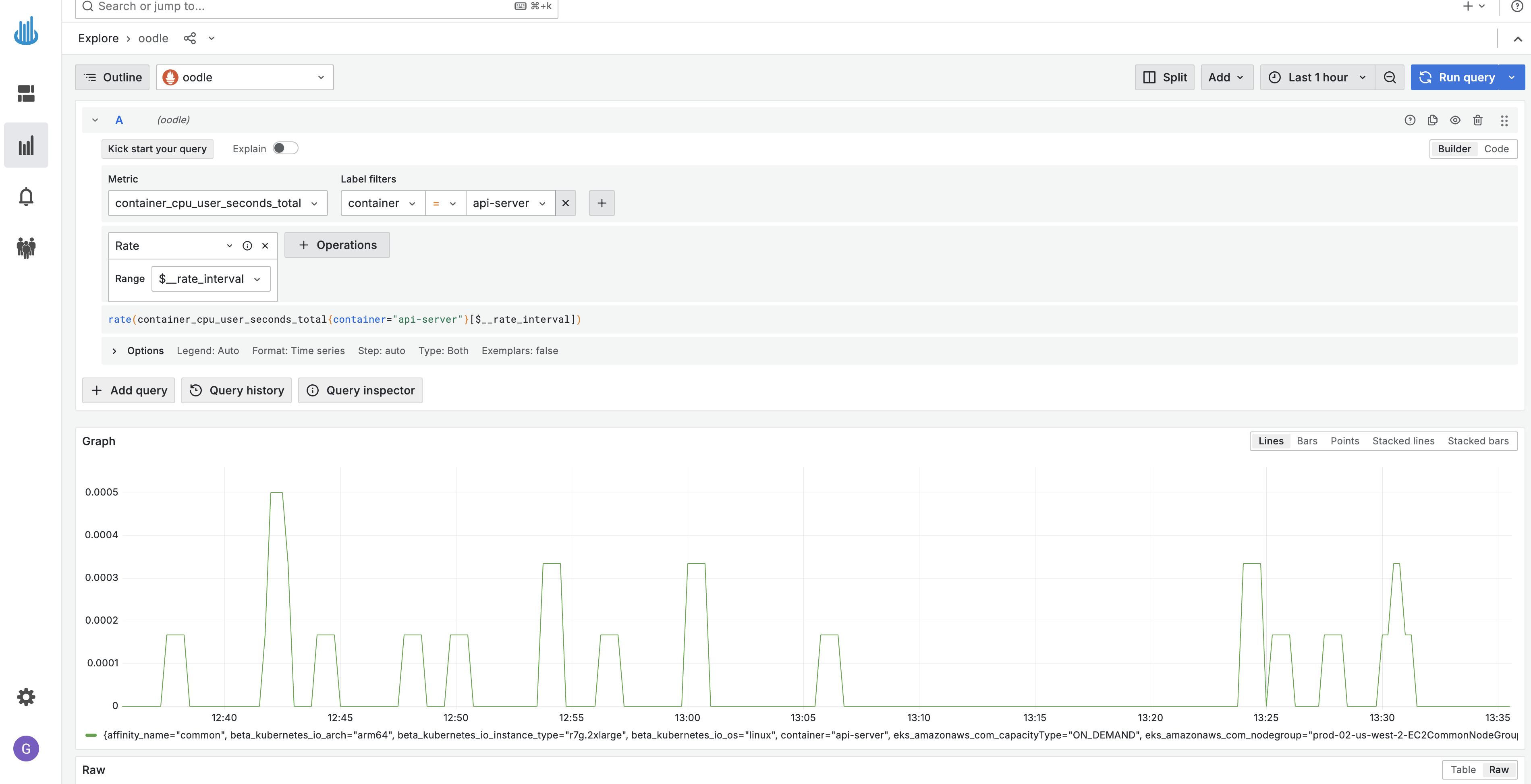
Support
If you have any questions or need any assistance, please contact us via our help chat app located at the bottom-right of the page or by reaching out to support@oodle.ai.